Report Logs for Android
This section covers how Instabug automatically attaches console logs, verbose logs, and all steps made by your users before a bug report is sent for Android apps.
Privacy Policy
It is highly recommended to mention in your privacy policy that you may be collecting logging data in order to assist troubleshooting bugs.
A variety of log types are sent with each crash or bug report. They appear within each report in your Instabug dashboard, as shown below. Log collection stops when Instabug is shown.
We support the following types of logs:
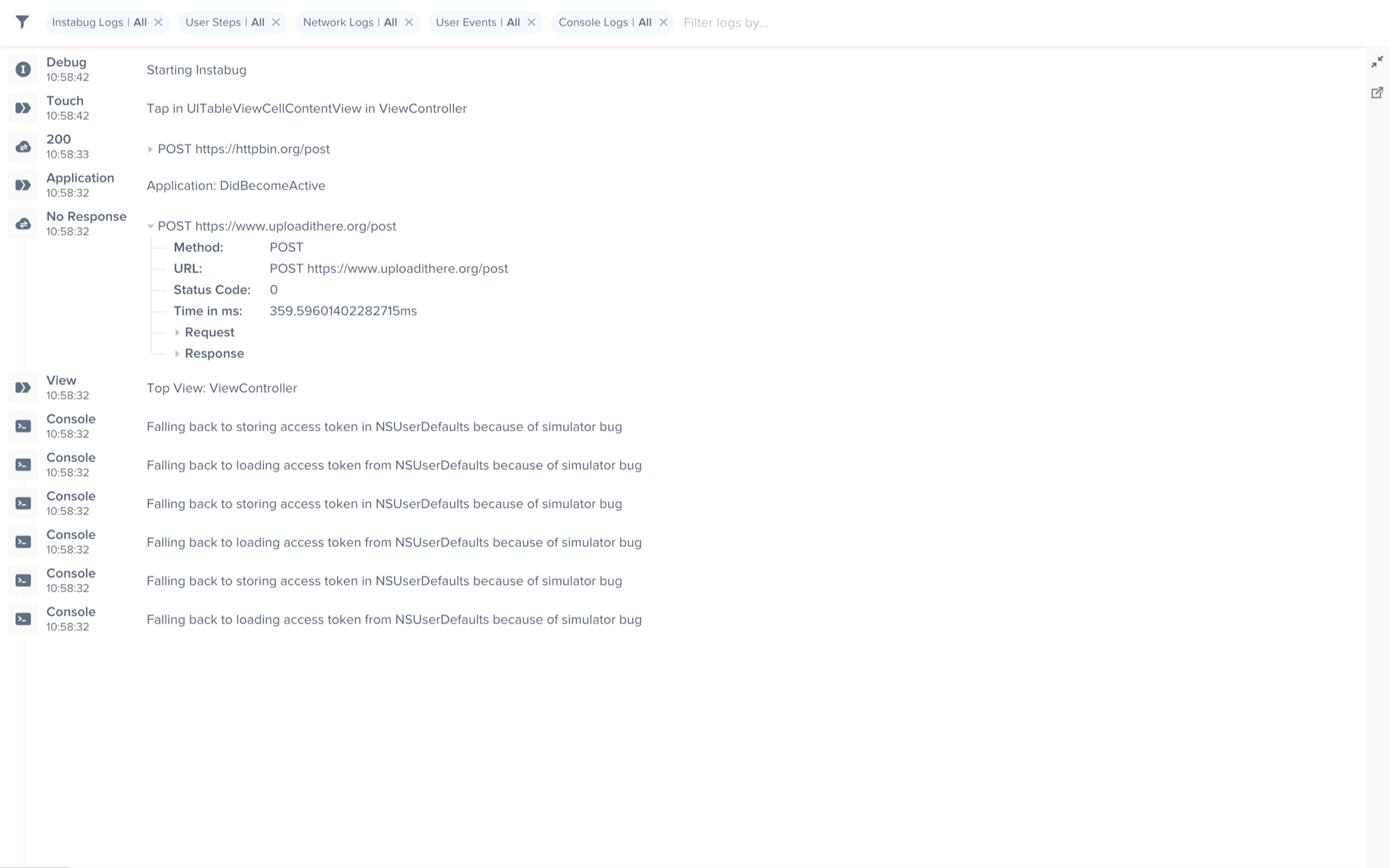
An example of the expanded logs view from your dashboard.
User Steps
Instabug can help you reproduce issues by tracking each step a user has taken until a report is sent. Note that the maximum number of user steps sent with each report is 100.
User Steps are formatted as follows: Event in text/viewID
of type class
in parentView
.
- The type of events captured are tap, double tap, long press, swipe, scroll and pinch.
text/viewID
refers to the text or the ID of the object that contains the event.Class
refers to the class of the object that contains the event.ParentView
refers to the view that contained the event.
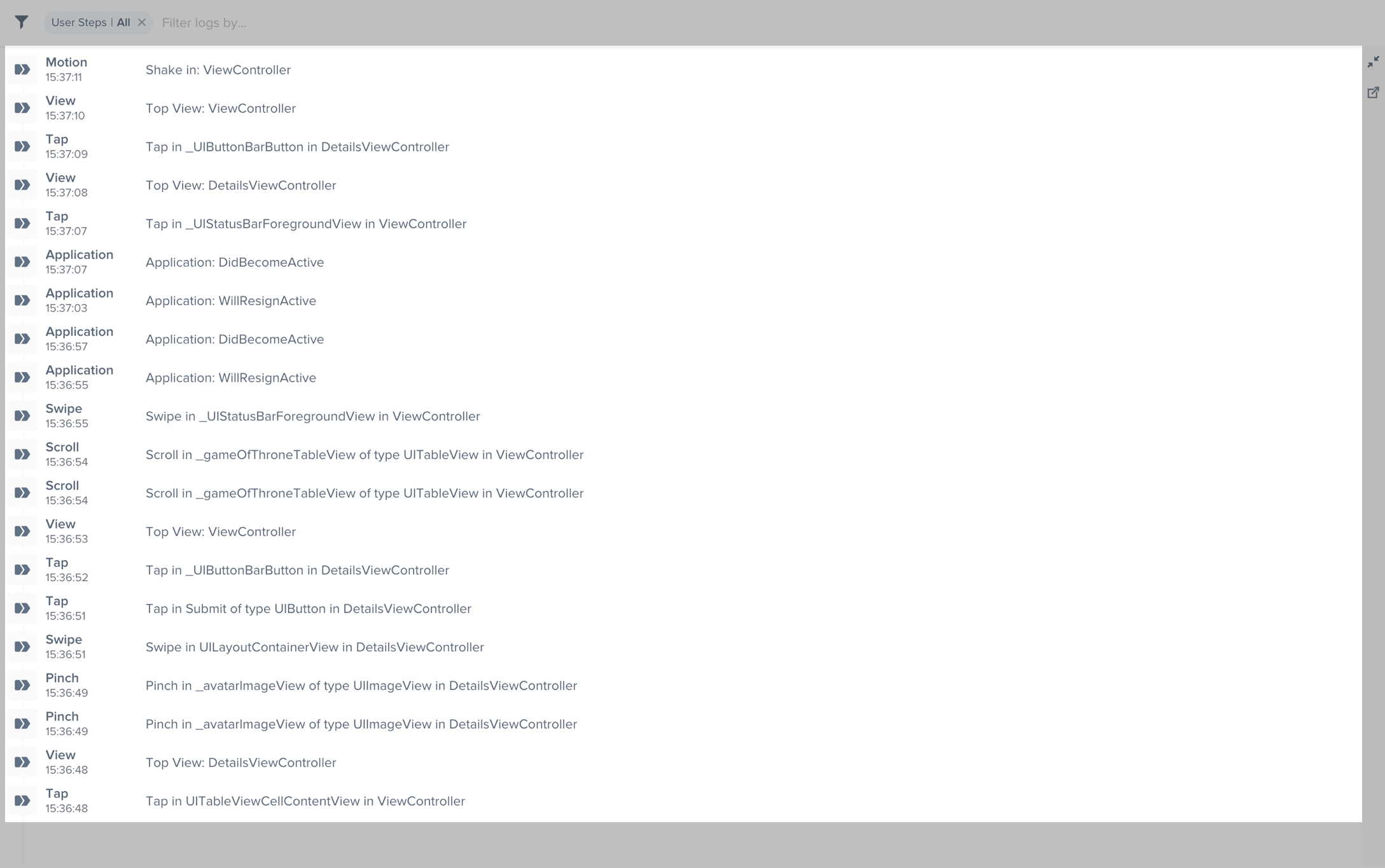
An example of the expanded logs view filtered by User Steps.
User steps collection can be disabled by using the following API:
Instabug.setTrackingUserStepsState(Feature.State.DISABLED)
Instabug.setTrackingUserStepsState(Feature.State.DISABLED);
Network Logs
Instabug automatically logs all network requests performed by your app from the start of the session. Requests details, along with their responses, are sent with each report. Instabug will also show you an alert at the top of the bug report in your dashboard when network requests have timed-out or taken too long to complete. Note that the maximum number of network logs sent with each report is 100.
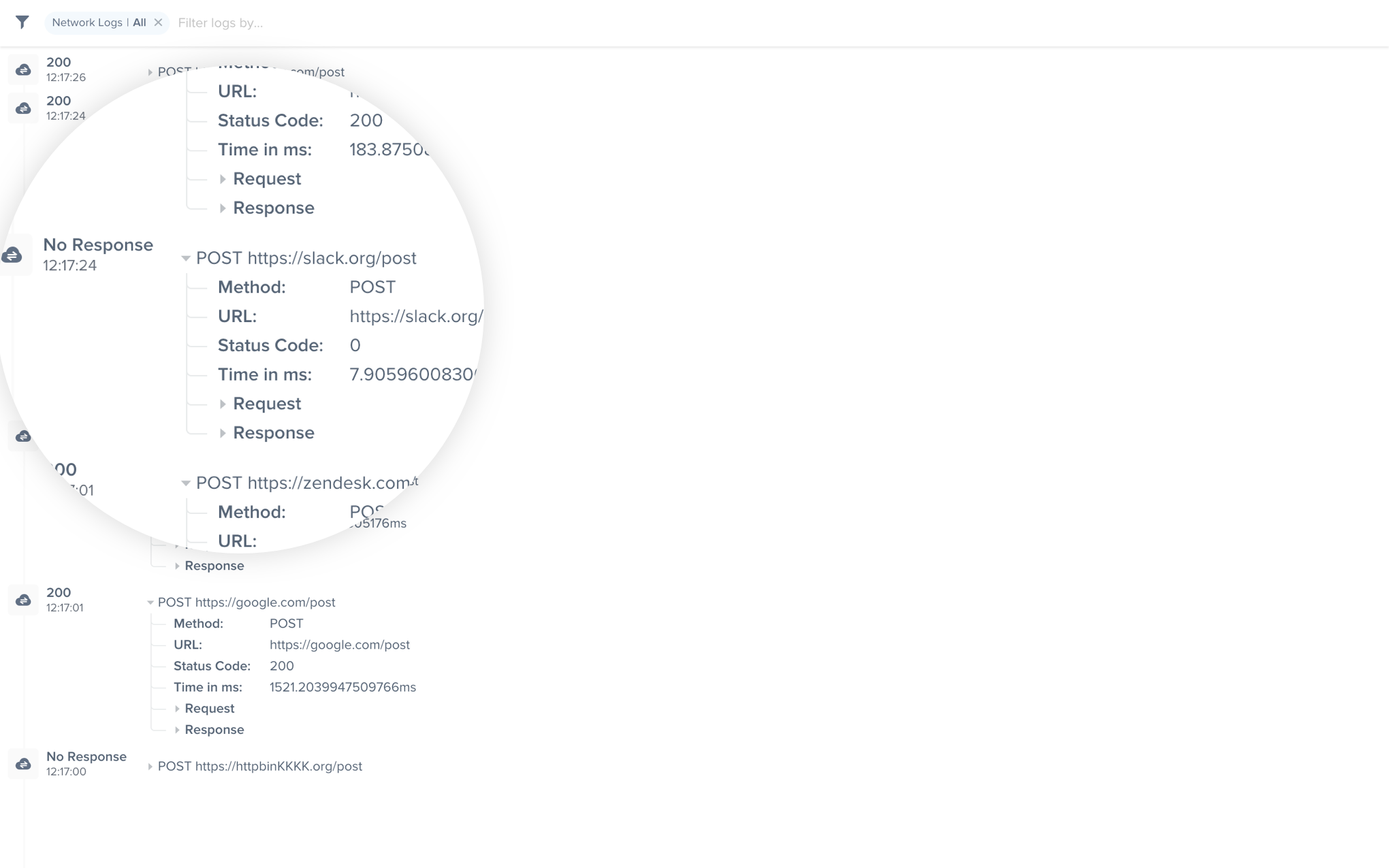
An example of network request logs in the Instabug dashboard.
Logging HttpUrlConnection
requests
HttpUrlConnection
requestsTo log network requests, use InstabugNetworkLog
then use the following method at the HttpUrlConnection
, requestBody
and responseBody
.
InstabugNetworkLog networkLog = new InstabugNetworkLog()
networkLog.Log(urlConnection, requestBody, responseBody)
InstabugNetworkLog networkLog = new InstabugNetworkLog();
networkLog.Log(urlConnection, requestBody, responseBody);
For a more detailed example, see the following network request.
@Override
protected String doInBackground(Void... params) {
HttpURLConnection urlConnection = null;
BufferedReader reader = null;
String moviesJsonStr = null;
try {
URL url = new URL("<YOUR_URL>");
urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setUseCaches(false);
urlConnection.setConnectTimeout(10000);
urlConnection.setReadTimeout(10000);
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.connect();
JSONObject jsonParam = new JSONObject();
try {
jsonParam.put("PARAM_1", "one");
jsonParam.put("PARAM_2", "two");
} catch (JSONException e) {
e.printStackTrace();
}
OutputStreamWriter out = new OutputStreamWriter(urlConnection.getOutputStream());
out.write(jsonParam.toString());
out.close();
InputStream inputStream = urlConnection.getInputStream();
StringBuffer buffer = new StringBuffer();
if (inputStream == null) {
return null;
}
reader = new BufferedReader(new InputStreamReader(inputStream));
String line;
while ((line = reader.readLine()) != null) {
buffer.append(line + "\n");
}
if (buffer.length() == 0) {
// Stream was empty. No point in parsing.
return null;
}
moviesJsonStr = buffer.toString();
//logging network request to instabug
InstabugNetworkLog networkLog = new InstabugNetworkLog();
networkLog.Log(urlConnection, jsonParam.toString(), moviesJsonStr);
} catch(Exception e) {
e.printStackTrace();
}
return moviesJsonStr;
}
Logging Okhttp requests
First, you will need to compile Instabug with a network interceptor.
implementation 'com.instabug.library:instabug-with-okhttp-interceptor:14.0.0'
To log Oktthp requests, use the InstabugOkhttpInterceptor
as shown in the following example.
val instabugOkhttpInterceptor = InstabugOkhttpInterceptor()
val client = OkHttpClient.Builder()
.addInterceptor(interceptor)
.addInterceptor(instabugOkhttpInterceptor)
.build()
InstabugOkhttpInterceptor instabugOkhttpInterceptor = new InstabugOkhttpInterceptor();
OkHttpClient client = new OkHttpClient.Builder()
.addInterceptor(interceptor)
.addInterceptor(instabugOkhttpInterceptor)
.build();
Modifying Requests
In the event that you need to modify a network request prior to sending it to the dashboard, you can follow the below steps:
1- Create a NetworkLogListener
object and modify the captured network log as shown below.
val networkLogListener = NetworkLogListener { networkLog: NetworkLogSnapshot ->
//Modify the received networkLog parameter
return@NetworkLogListener networkLog
}
NetworkLogListener networkLogListener = new NetworkLogListener() {
@Override
public NetworkLogSnapshot onNetworkLogCaptured(NetworkLogSnapshot networkLog) {
//Modify the received networkLog parameter
return networkLog;
// To exclude the network trace from being captured return null
// return null;
}
};
2- Register the created NetworkLogListener
to your InstabugOkHttpInterceptor
object. This can be done through two different methods:
a. Pass it in the constructor.
val instabugOkhttpInterceptor = InstabugOkhttpInterceptor(networkLogListener)
b. Call registerNetworkLogsListener
method on InstabugOkhttpInterceptor
object.
val instabugOkhttpInterceptor = InstabugOkhttpInterceptor()
instabugOkhttpInterceptor.registerNetworkLogsListener(networkLogListener)
// For the modifications to reflect in APM as well, you can use the below network logs listener
APM.registerNetworkLogsListener(networkLogListener);
In case you need to remove the network listener, you can use the below method:
instabugOkhttpInterceptor.removeNetworkLogsListener()
// Use the below API to remove APM's network logs listener
APM.registerNetworkLogsListener(null)
instabugOkhttpInterceptor.removeNetworkLogsListener();
// Use the below API to remove APM's network logs listener
APM.registerNetworkLogsListener(null)
Instabug Logs
You can log messages throughout your application's lifecycle to be sent with each report. InstabugLog
works just like the regular Log
class you use to show colorful logs in your logcat. Note that the maximum number of Instabug logs sent with each report is 1,000.
InstabugLog.d("Message to log")
InstabugLog.v("Message to log")
InstabugLog.i("Message to log")
InstabugLog.e("Message to log")
InstabugLog.w("Message to log")
InstabugLog.wtf("Message to log")
InstabugLog.d("Message to log");
InstabugLog.v("Message to log");
InstabugLog.i("Message to log");
InstabugLog.e("Message to log");
InstabugLog.w("Message to log");
InstabugLog.wtf("Message to log");
Console Logs
Instabug captures all console logs and displays them on your dashboard with each report. Note that the maximum number of console logs sent with each report is 1,000 statements with a limit of 5,000 characters for each statement.
To disable console logs.
Instabug.Builder(this, "token")
.setConsoleLogState(Feature.State.DISABLED)
.build()
new Instabug.Builder(this, "token")
.setConsoleLogState(Feature.State.DISABLED)
.build();
User Events
Best Practices
Currently the limit of the number of user events sent with each report is 1,000. If you're planning on logging a large amount of unique data, the best practice here would be to use Instabug Logging instead. The reason for this is that having a very large amount of user events will negatively impact the performance of the dashboard.
Having a large amount of user events will not affect dashboard performance if the user events are not unique.
You can log custom user events throughout your application and they will automatically be included with each report. Note that the maximum number of user events sent with each report is 1,000.
Instabug.logUserEvent("Logged in")
Instabug.logUserEvent("Logged in");
Updated 2 months ago
Logs go hand-in-hand with both bug and crash reporting, so why not give those a look? The Session Profiler feature also give you more comprehensive details alongside logs.