Setting Custom Data for iOS
Discussed here is how to set user attributes and tags, as well as log user events, and their relevant APIs for your iOS app.
User Attributes
You can assign custom attributes to your users and they will show up on your Instabug dashboard with each report. These attributes can later be used to filter reports in your dashboard.
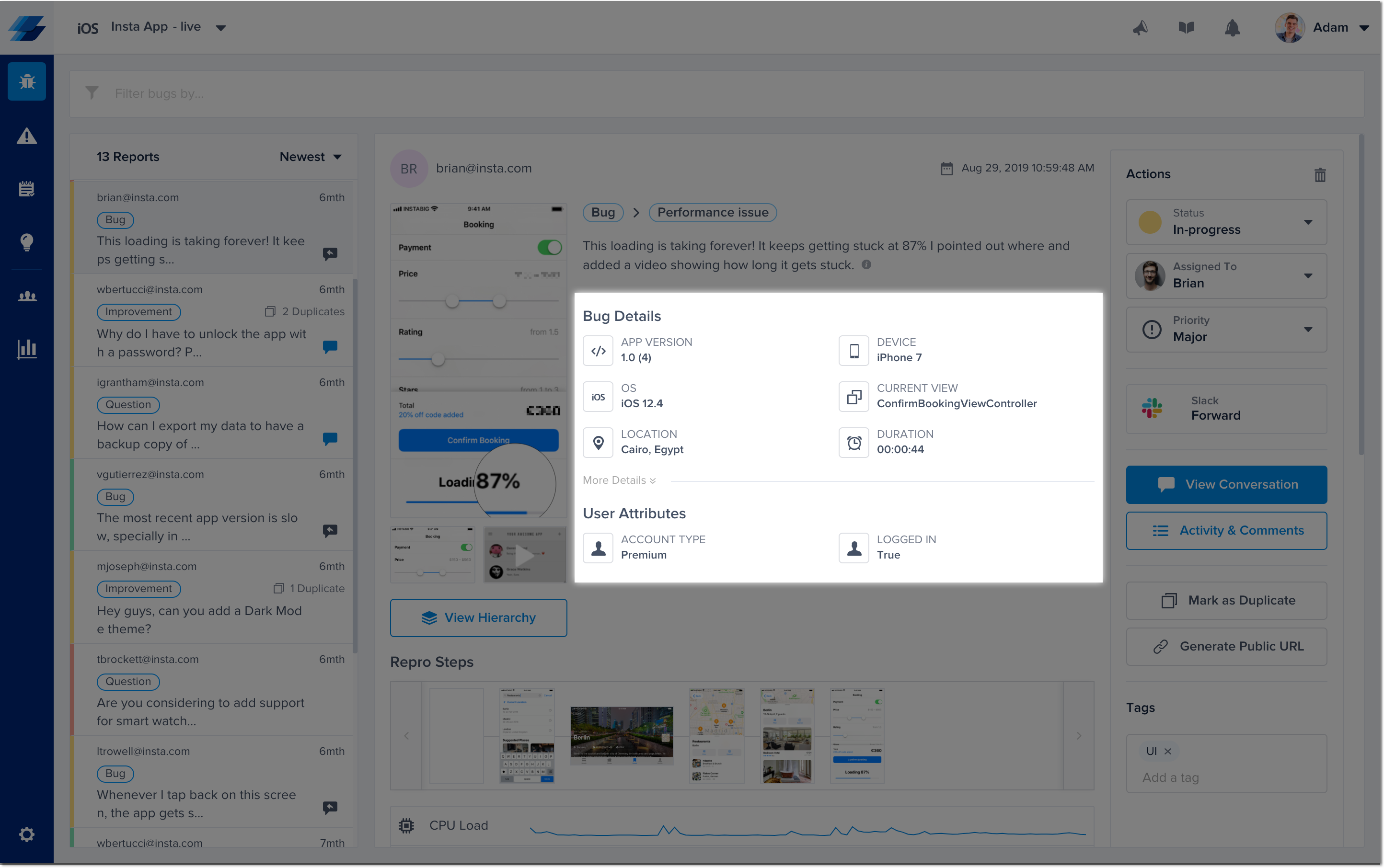
This is where additional user attributes appear in your bug reports.
To add a new user attribute, use the following method.
Instabug.setUserAttribute("True", withKey: "Logged in")
Instabug.setUserAttribute("False", withKey: "Completed IAP")
[Instabug setUserAttribute:@"True" withKey:@"Logged in"];
[Instabug setUserAttribute:@"18" withKey:@"Age"];
You can also retrieve or remove the current value of a certain user attribute.
let loggedIn = Instabug.userAttribute(forKey: "Logged in")
Instabug.removeUserAttribute(forKey: "Logged in")
NSString *loggedIn = [Instabug userAttributeForKey:@"Logged in"];
[Instabug removeUserAttributeForKey:@"Logged in"];
Or retrieve all user attributes.
let allUserAttributes = Instabug.userAttributes()
NSDictionary *allUserAttributes = [Instabug userAttributes];
User Events
You can log custom user events throughout your application. Custom events are automatically included with each report.
Instabug.logUserEvent(withName: "Skipped Walkthrough")
[Instabug logUserEventWithName:@"Skipped Walkthrough"];
Tags
You can add custom tags to your bug and crash reports. These tags can later be used to filter reports or set custom rules from your dashboard.
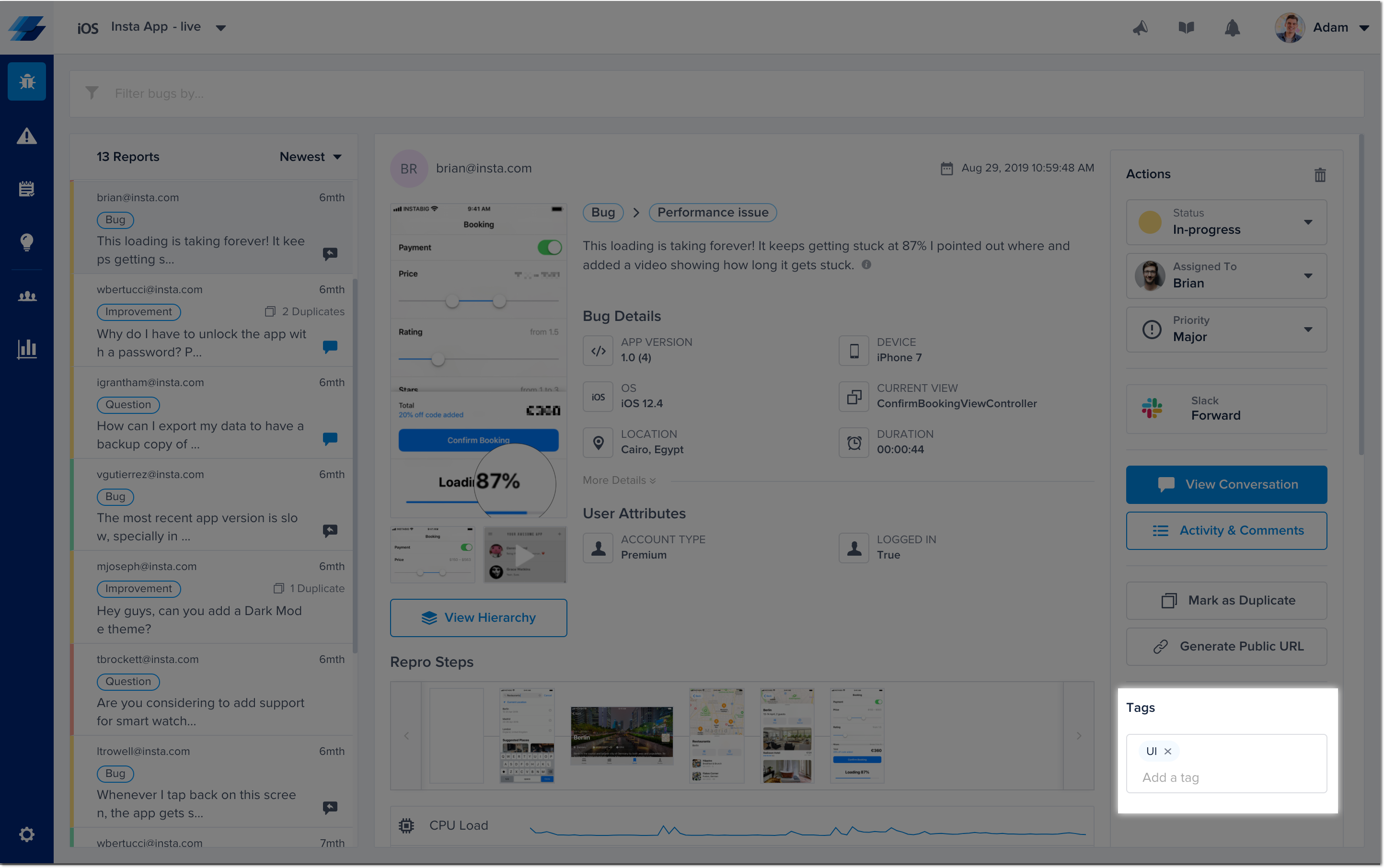
This is where tags appear in your bug reports.
The example below demonstrates how to add tags to a report.
Instabug.appendTags(["Design", "Flow"])
[Instabug appendTags:@[@"Design", @"Flow"]];
Adding tags before sending reports
Sometimes it's useful to be able to add a tag to a bug report before it's been sent. In these cases, the perfect solution would be use the event handlers of the bug reporting class. You can find more details here.
You can also get all the currently set tags as follows.
let tags = Instabug.getTags()
NSString *tags = [Instabug getTags];
Last, you can reset all the tags.
Instabug.resetTags()
[Instabug resetTags];
Managing Tags
If you'd like to remove a particular tag from your dashboard to prevent it from appearing again when entering a new tag manually, you can do so by navigating to the tags page under the settings options and remove the tag. You can also edit and rename the tag.
Experiments
Included in Crash Reporting and APM
This feature is currently available for the following metrics:
- Crash Occurrences.
- UI Hangs.
- Cold App Launches.
- Screen Loading.
In certain scenarios, you might find that you're rolling out different experiments to different users, where your user base would see different features depending on what's enabled for them. In scenarios such as these, you'll want to keep track of the enabled experiments for each user and even filter by them.
This is possible using the 'experiments' methods provided by the SDK. You can have up to 200 experiments, with no duplicates. Each experiment can consist of 70 characters at most and is not removed at the end of the session or if logOut
is called.
Add Experiment
You can use the below method to add experiments to the next report:
Instabug.addExperiments(["exp1"])
[Instabug addExperiments:@[@"exp1"]];
Remove Experiment
You can use the below method to remove certain experiments from the next report:
Instabug.removeExperiments(["exp1"])
[Instabug removeExperiments:@[@"exp2"]];
Clear Experiment
You can use the below method to clear experiments from the next report:
Instabug.clearAllExperiments()
[Instabug clearAllExperiments];
Updated 6 months ago
You now have more information than ever about each bug and crash report, so we suggest you read up more on bug reporting. Also, did you know that you can use custom user attributes and events to target surveys?