Report Logs for iOS
This section covers how Instabug automatically attaches console logs, verbose logs, and all steps made by your users before a bug report is sent for iOS apps.
Privacy Policy
It is highly recommended to mention in your privacy policy that you may be collecting logging data in order to assist with troubleshooting bugs.
A variety of log types are sent with each crash or bug report. They appear within each report in your Instabug dashboard, as shown below. Log collection stops when Instabug is shown.
We support the following types of logs:
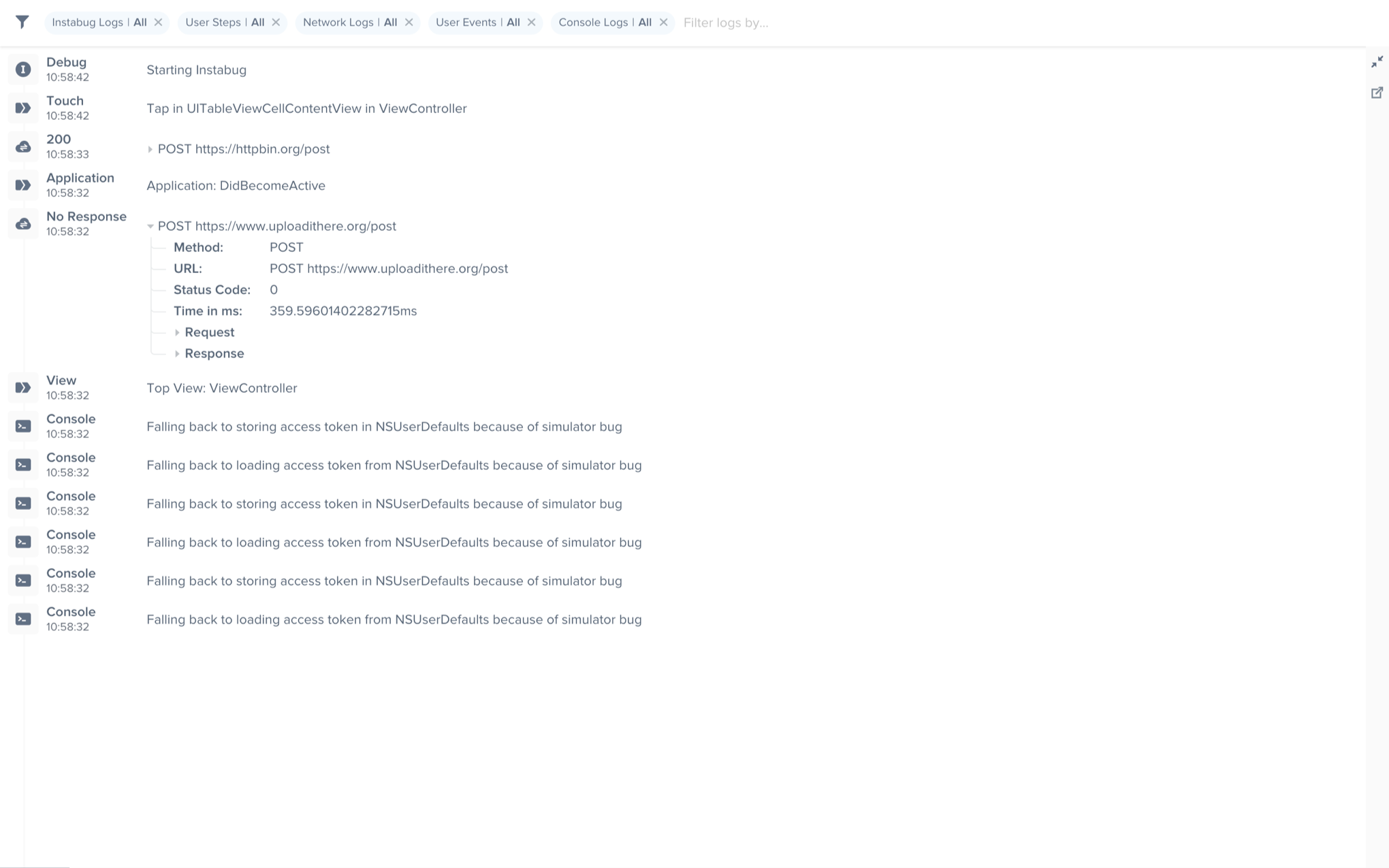
An example of the expanded logs view from your dashboard.
User Steps
Instabug can help you reproduce issues by tracking each step a user has taken until a report is sent. Note that the maximum number of user steps sent with each report is 100.
User Steps are formatted as follows: Event in label
of type class
in controller
For example: Tap in UITableViewCellContentView
in ViewController
- The type of events captured are tap, long press, force touch, swipe, scroll and pinch.
- We also capture life cycle events such as entering background, entering foreground, became active, resign active, and memory warning.
Label
refers to the label of the object that contains the event.Class
refers to the class of the object that contains the event.Controller
refers to the view that contained the event.
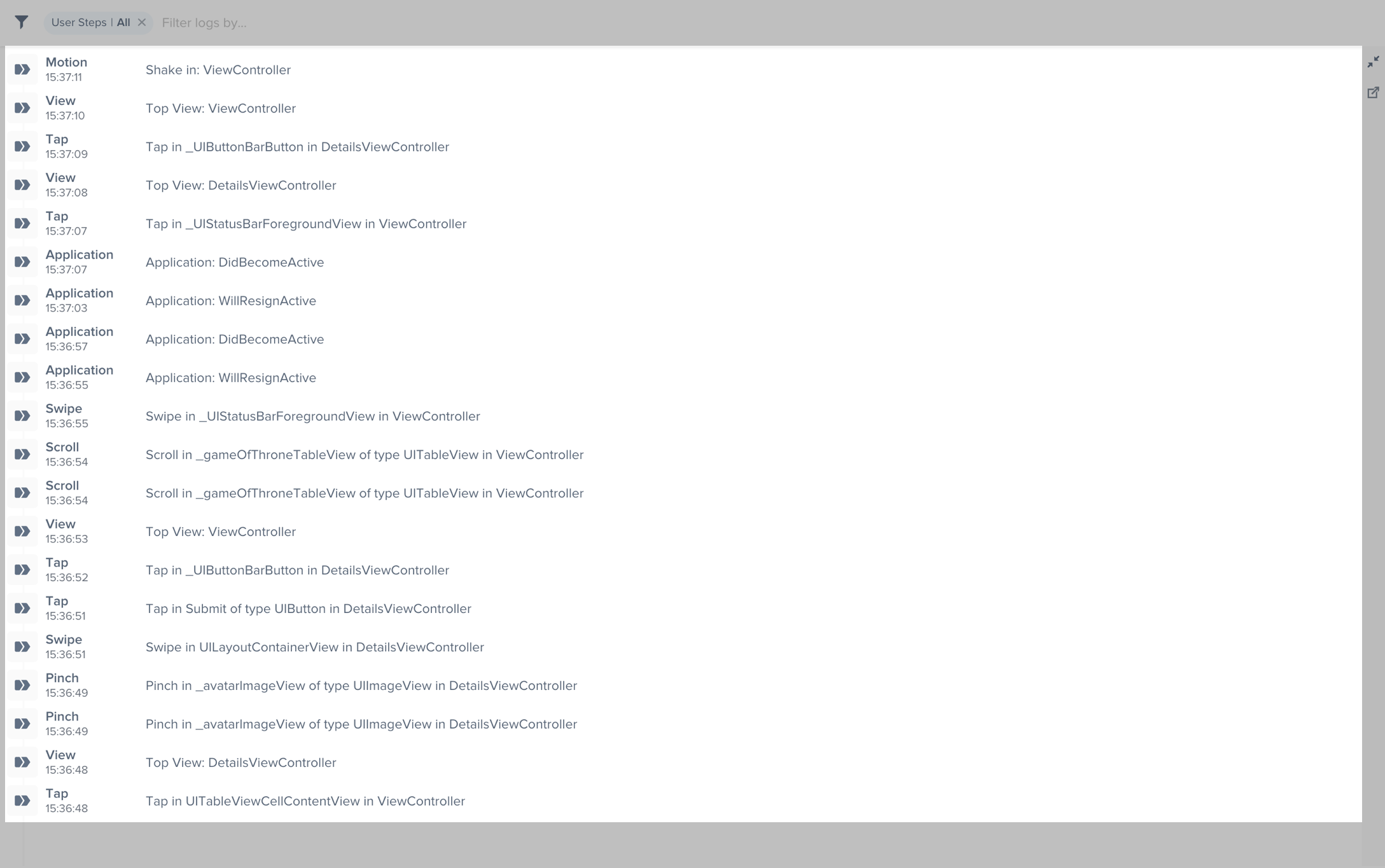
An example of the expanded logs view filtered by User Steps.
You can disable User Steps with the following method.
Instabug.trackUserSteps = false
Instabug.trackUserSteps = NO;
To be able to capture User Steps, we need to do method swizzling. We take an approach to swizzling that is absolutely safe and does not impact your app negatively in any way. For more details, you can check here. This swizzling can be disabled if you would prefer to log the user interactions manually.
Network Logs
Instabug automatically logs all network requests performed by your app from the start of the session. Requests details, along with their responses, are sent with each report. Instabug will also show you an alert at the top of the bug report in your dashboard when network requests have timed-out or taken too long to complete. Note that the maximum number of network logs sent with each report is 1,000.
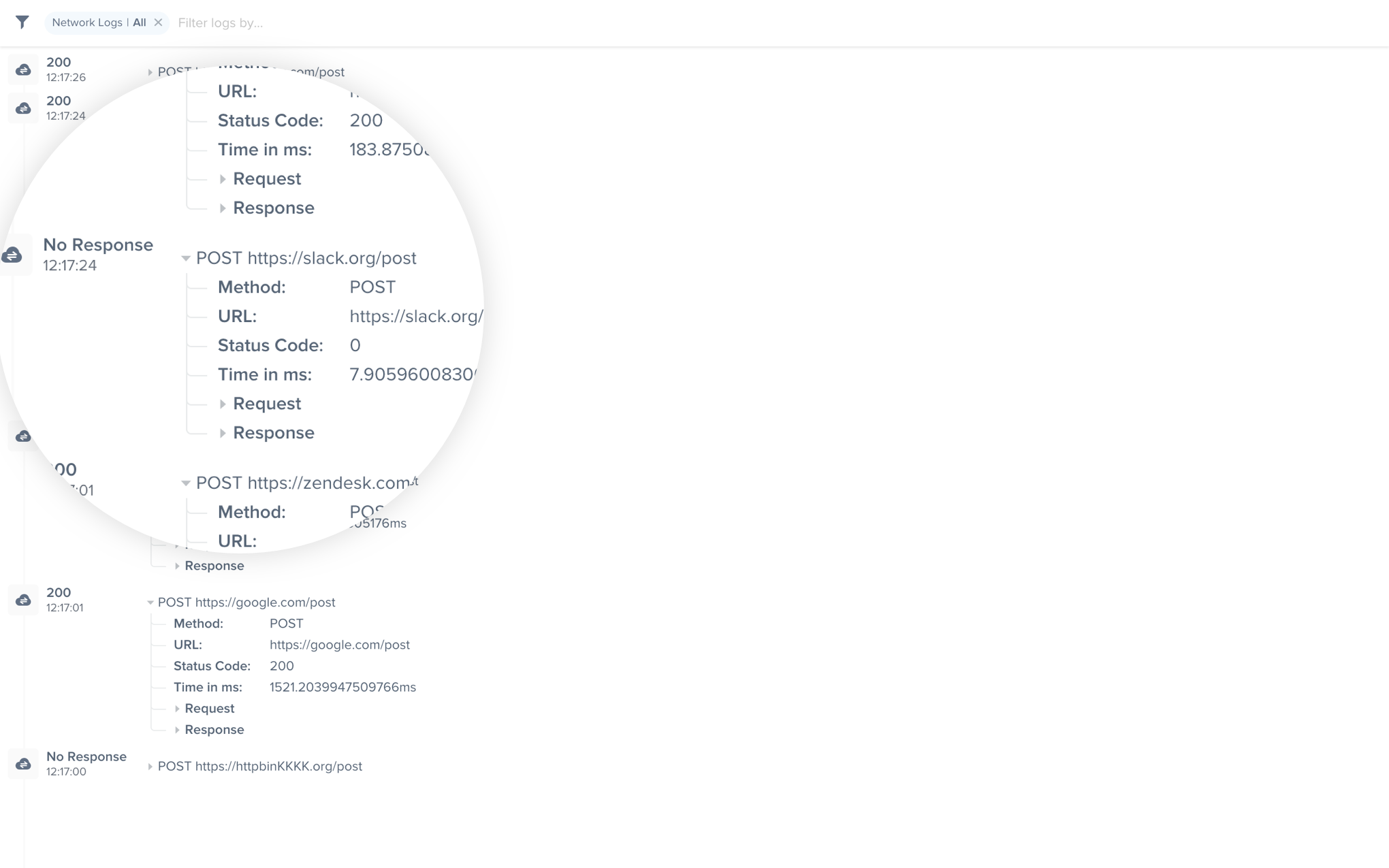
An example of network request logs in the Instabug dashboard.
Omitting Requests
You can omit requests from being logged based on either their request or response details.
+ [Instabug setNetworkLoggingRequestFilterPredicate:responseFilterPredicate:]
allows you to specify two predicates to be evaluated against every request and response to determine if the request should be included in logs or not.
let path = "/products"
let requestPredicate = NSPredicate(format: "URL.path MATCHES %@", path)
let responsePredicate = NSPredicate(format: "statusCode >= %d AND statusCode <= %d", 200, 399)
NetworkLogger.setNetworkLoggingRequestFilterPredicate(requestPredicate, responseFilterPredicate: responsePredicate)
NSString *path = @"/products";
NSPredicate *requestPredicate = [NSPredicate predicateWithFormat:@"URL.path MATCHES %@", path];
NSPredicate *responsePredicate = [NSPredicate predicateWithFormat:@"statusCode >= %d AND statusCode <= %d", 200, 399];
[IBGNetworkLogger setNetworkLoggingRequestFilterPredicate:requestPredicate responseFilterPredicate:responsePredicate];
The code above excludes all requests made to URLs that have /products
path. It also excludes all responses that have a success and redirection status code, thus only including requests with 4xx and 5xx responses.
requestFilterPredicate
is evaluated against an NSURLRequest
, while responseFilterPredicate
is evaluated against an NSHTTPURLResponse
.
Obfuscating Data
Requests
You can obfuscate sensitive user data in requests, like authentication tokens for example, without filtering out the whole request.
NetworkLogger.setRequestObfuscationHandler { (request) -> URLRequest in
var myRequest:NSMutableURLRequest = request as! NSMutableURLRequest
let urlString = request.url?.absoluteString
urlString = obfuscateAuthenticationTokenInString()
let obfuscatedURL = URL(string: urlString)
myRequest.url = obfuscatedURL
return myRequest.copy() as! URLRequest
}
IBGNetworkLogger.requestObfuscationHandler = ^NSURLRequest * _Nonnull(NSURLRequest * _Nonnull request) {
NSMutableURLRequest *myRequest = [request mutableCopy];
NSString *urlString = request.URL.absoluteString;
urlString = [self obfuscateAuthenticationTokenInString:urlString];
NSURL *obfuscatedURL = [NSURL URLWithString:urlString];
myRequest.URL = obfuscatedURL;
return myRequest;
};
Responses
As with requests, the response object, as well as the response data, can be modified for obfuscation purposes before they are logged.
NetworkLogger.setResponseObfuscationHandler { (data, response, completion) in
if let data = data {
let modifiedData = self.modify(data: data)
let modifiedResponse = self.modify(response: response)
completion(modifiedData, modifiedResponse)
}
}
[IBGNetworkLogger setResponseObfuscationHandler:^(NSData * _Nullable responseData, NSURLResponse * _Nonnull response, NetworkObfuscationCompletionBlock _Nonnull returnBlock) {
NSData *modifiedData = [self obfuscateData:responseData];
NSURLResponse *modifiedResponse = [self obfuscateResponse:response];
returnBlock(modifiedData, modifiedResponse);
}];
Requests Not Appearing
If your network requests aren't being logged automatically, that probably means you're using a custom NSURLSession
or NSURLSessionConfiguration
.
To enable logging for your NSURLSession
, add the following code.
let configuration = URLSessionConfiguration.ephemeral
NetworkLogger.enableLogging(for: configuration)
let session = URLSession(configuration: configuration)
NSURLSessionConfiguration *configuration = NSURLSessionConfiguration.ephemeralSessionConfiguration;
[IBGNetworkLogger enableLoggingForURLSessionConfiguration:configuration];
NSURLSession *session = [NSURLSession sessionWithConfiguration:configuration];
If the requests still aren't appearing
You'll need to make sure that
enableLoggingForURLSessionConfiguration:
was called just before using the configuration to create the session.
AFNetworking
To enable logging for AFNetworking, create the following class.
// IBGAFURLSessionManager.h
#import <AFNetworking/AFNetworking.h>
@interface IBGAFURLSessionManager : AFURLSessionManager
@end
// IBGAFURLSessionManager.m
#import "IBGAFURLSessionManager.h"
#import <Instabug/Instabug.h>
@implementation IBGAFURLSessionManager
- (instancetype)initWithSessionConfiguration:(nullable NSURLSessionConfiguration *)configuration {
[IBGNetworkLogger enableLoggingForURLSessionConfiguration:configuration];
return [super initWithSessionConfiguration:configuration];
}
@end
Then use IBGAFURLSessionManager
to create your requests.
Alamofire
To enable logging for Alamofire, create the following class.
import Alamofire
import Instabug
class IBGSessionManager: Alamofire.Session {
static let sharedManager: IBGSessionManager = {
let configuration = URLSessionConfiguration.default
NetworkLogger.enableLogging(for: configuration)
let manager = IBGSessionManager(configuration: configuration)
return manager
}()
}
Then use IBGSessionManager
to create your requests.
Implementing SSL pinning
If you use SSL pinning, you'll need to call our APIs as the SDK takes care of intercepting and making the request in order to log it. The following code snippet should help with doing so.
NetworkLogger.setCanAuthenticateAgainstProtectionSpaceHandler { (_) -> Bool in
return true
}
NetworkLogger.setDidReceiveAuthenticationChallengeHandler { (challenge) -> URLCredential? in
guard let trust = challenge.protectionSpace.serverTrust else {
return nil
}
return URLCredential(trust: trust)
}
[IBGNetworkLogger setCanAuthenticateAgainstProtectionSpaceHandler:^BOOL(NSURLProtectionSpace * _Nonnull protectionSpace) {
return YES;
}];
[IBGNetworkLogger setDidReceiveAuthenticationChallengeHandler:^NSURLCredential * _Nullable(NSURLAuthenticationChallenge * _Nonnull challenge) {
SecTrustRef trust = challenge.protectionSpace.serverTrust;
if (trust == nil) {
return nil;
}
return [NSURLCredential credentialForTrust:trust];
}];
Disabling Requests
Network request logging is enabled by default if it's included in your plan. To disable it, use the following method.
NetworkLogger.enabled = false
IBGNetworkLogger.enabled = NO;
Instabug Logs
Instabug Logs are similar to NSLog()
and print()
, but they have the added benefit of having different verbosity levels. This lets you filter logs based on their verbosity level when viewing a report on your Instabug dashboard. Note that the maximum number of Instabug logs sent with each report is 1,000.
Use Instabug Logs through the following methods.
IBGLog.log("Log statement")
IBGLog.logVerbose("Verbose statement")
IBGLog.logInfo("Info statement")
IBGLog.logWarn("Warning statement")
IBGLog.logDebug("Debug statement")
IBGLog.logError("Error statement")
IBGLog(@"Log message");
IBGLogVerbose(@"Verbose log message");
IBGLogDebug(@"Debug log message");
IBGLogInfo(@"Info log message");
IBGLogWarn(@"Warn log message");
IBGLogError(@"Error log message");
Instabug Logs with 3rd Party Loggers
If you use CocoaLumberjack or XCGLogger, you can easily route all your logs to Instabug using our log destinations. Check the instructions on GitHub for CocoaLumberjack and XCGLogger.
Console Logs
Instabug captures all console logs and displays them on your dashboard with each report. Note that the maximum number of console logs sent with each report is 500 statements with an unlimited number of characters for each statement.
Console Logs on iOS 10
Due to the changes that Apple has made to how logging works, we can only capture console logs on iOS versions prior to 10.
To work around this issue, you can add the following snippet to your AppDelegate
file but outside the scope of the class to allow Instabug to capture logs automatically on iOS 10 and above.
inline void NSLog(NSString *format, ...) {
va_list arg_list;
va_start(arg_list, format);
IBGNSLogWithLevel(format, arg_list, IBGLogLevelDefault);
va_end(arg_list);
}
Alternatively, you can replace all your NSLog()
and print()
statements with IBGLog
.
Another workaround in case you are using Swift 3.0 or above is to send console logs through the following print
method. Make sure to define this method outside the AppDelegate
class scope in the correct place such that it becomes accessible in all your classes.
public func print(_ items: Any..., separator: String = " ", terminator: String = "\n") {
let output = items.map { "\($0)" }.joined(separator: separator)
Swift.print(output, terminator: terminator);
IBGLog.log(output)
}
User Events
Best Practices
Currently the limit of the number of user events sent with each report is 1,000. If you're planning on logging a large amount of unique data, the best practice here would be to use Instabug Logging instead. The reason for this is that having a very large amount of user events will negatively impact the performance of the dashboard.
Having a large amount of user events will not affect dashboard performance if the user events are not unique.
You can log custom user events throughout your application and they will automatically be included with each report. Note that the maximum number of user events sent with each report is 1,000.
Instabug.logUserEvent(withName: "Skipped Walkthrough")
[Instabug logUserEventWithName:@"Skipped Walkthrough"];
Updated 6 months ago
Logs go hand-in-hand with both bug and crash reporting, so why not give those a look? The Session Profiler feature also give you more comprehensive details alongside logs.